买服务器上雨云
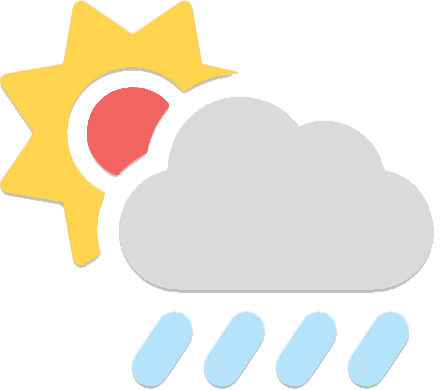
import iconv from 'iconv';
import axios from 'axios';
const resp = await axios.get(url, {
responseType: 'arraybuffer', // node环境
});
return iconv.decode(resp.data, 'gb2312');
import axios from 'axios';
const config = {
method: 'POST',
url: 'https://www.moedm.net/index.php/api/vod',
headers: {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/126.0.0.0 Safari/537.36 Edg/126.0.0.0',
},
rejectUnauthorized:false, //关闭证书校验
data: {}
};
const resp = await axios(config)
const code = '[1,3,4]'; // typeof string
const jsonCode = new Function(`return ${code}`)(); // typeof object
常用于提取演员数据
const html = `
<div class="persons">
<div class="person" itemprop="actor" itemscope="" itemtype="http://schema.org/Person">
<meta itemprop="name" content="Elijah Wood">
<div class="img">
<a href="https://www.4kvm.org/cast/elijah-wood"><img alt="Elijah Wood isFrodo" src="https://gimg0.baidu.com/gimg/app=2001&n=0&g=0n&fmt=jpeg&src=image.tmdb.org/t/p/w92/7UKRbJBNG7mxBl2QQc5XsAh6F8B.jpg"></a>
</div>
<div class="data">
<div class="name"><a itemprop="url" href="https://www.4kvm.org/cast/elijah-wood">Elijah Wood</a></div>
<div class="caracter">Frodo</div>
</div>
</div>
<div class="person" itemprop="actor" itemscope="" itemtype="http://schema.org/Person">
<meta itemprop="name" content="Ian McKellen">
<div class="img">
<a href="https://www.4kvm.org/cast/ian-mckellen"><img alt="Ian McKellen isGandalf" src="https://gimg0.baidu.com/gimg/app=2001&n=0&g=0n&fmt=jpeg&src=image.tmdb.org/t/p/w92/5cnnnpnJG6TiYUSS7qgJheUZgnv.jpg"></a>
</div>
<div class="data">
<div class="name"><a itemprop="url" href="https://www.4kvm.org/cast/ian-mckellen">Ian McKellen</a></div>
<div class="caracter">Gandalf</div>
</div>
</div>
</div>
`;
pdfa(html, ".persons .person")
.map((item) => pdfh(item, "meta&&content"))
.join(","),
常用于截取内容后是一段代码串, 但不想使用正则提取
const html = `var name = "zyplayer"; name = "zyfun";`;
eval(html);
console.log(name);
常用于加密代码执行补充环境且避免污染全局变量
// 加密代码
const code = function() {
var _0x30e6a9 = "e11ed29b";
function _0x52e1af(_0x174b0c, _0x4fda13) {
var _0x550aaa = atob(_0x174b0c);
for (var _0x488b1e, _0x4bd62c = [], _0x11662e = 0, _0x27b014 = "", _0x428133 = 0; 256 > _0x428133; _0x428133++) {
_0x4bd62c[_0x428133] = _0x428133;
}
for (_0x428133 = 0; 256 > _0x428133; _0x428133++) {
_0x11662e = (_0x11662e + _0x4bd62c[_0x428133] + _0x4fda13.charCodeAt(_0x428133 % _0x4fda13.length)) % 256;
_0x488b1e = _0x4bd62c[_0x428133];
_0x4bd62c[_0x428133] = _0x4bd62c[_0x11662e];
_0x4bd62c[_0x11662e] = _0x488b1e;
}
for (b = _0x11662e = _0x428133 = 0; b < _0x550aaa.length; b++) {
_0x428133 = (_0x428133 + 1) % 256;
_0x11662e = (_0x11662e + _0x4bd62c[_0x428133]) % 256;
_0x488b1e = _0x4bd62c[_0x428133];
_0x4bd62c[_0x428133] = _0x4bd62c[_0x11662e];
_0x4bd62c[_0x11662e] = _0x488b1e;
_0x27b014 += String.fromCharCode(_0x550aaa.charCodeAt(b) ^ _0x4bd62c[(_0x4bd62c[_0x428133] + _0x4bd62c[_0x11662e]) % 256]);
}
return _0x27b014;
}
if (!JSON.decrypt || typeof JSON.decrypt !== "function") {
Object.defineProperty(JSON, "decrypt", {
"value": function (_0x58f264) {
var _0x2da9b8 = _0x52e1af(_0x58f264, _0x30e6a9);
return this.parse(_0x2da9b8);
}
});
}
}
(code)(); // 执行后JSON对象会挂载decrypt方法
console.log(JSON);
// 毫秒级时间戳(13位)
new Date().getTime();
new Date().valueOf();
Date.now();
+new Date();
// 秒级时间戳(10位)
Math.floor(new Date().getTime() / 1000);
常用于生成唯一值id, 如下为三种算法, 可选其一
const generateUUID = () => {
if (crypto && typeof crypto.randomUUID === "function") {
// 算法一: randomUUID
return crypto.randomUUID();
} else if (crypto && typeof crypto.getRandomValues === "function") {
// 算法二: getRandomValues填充
return "10000000-1000-4000-8000-100000000000".replace(/[018]/g, (c) =>
(
+c ^
(crypto.getRandomValues(new Uint8Array(1))[0] & (15 >> (+c / 4)))
).toString(16)
);
} else {
// 算法三: Math.random填充
return "xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx".replace(
/[xy]/g,
function (c) {
const r = (Math.random() * 16) | 0,
v = c == "x" ? r : (r & 0x3) | 0x8;
return v.toString(16);
}
);
}
}